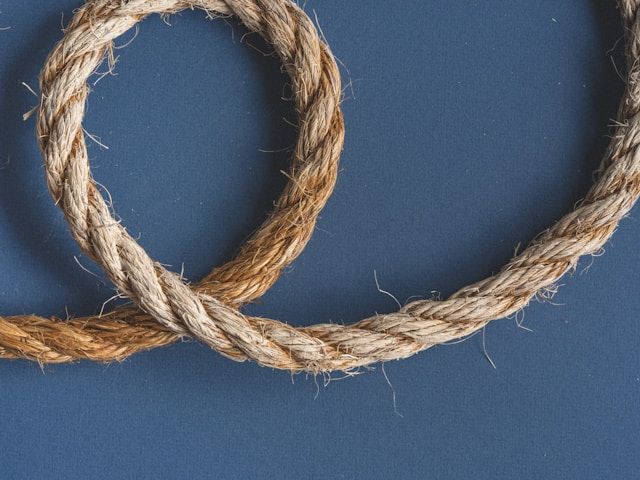
How JavaScript Event Loop works
The JavaScript Event Loop Explained
If you've spent much time working with JavaScript, you've likely heard the term "event loop" thrown around. But what exactly is the event loop and why is it important? The event loop is a key concept for understanding how JavaScript executes code in a non-blocking way.
JavaScript is a single-threaded language, which means it can only execute one piece of code at a time. This seems limiting, since typical programs involve many different tasks happening concurrently like making network requests, handling user input, updating the DOM, etc. The event loop is what allows JavaScript to handle concurrent operations.
The Event Loop
The event loop continually checks the call stack to see if there are any functions to run. If the stack is empty, it checks the message queue to see if there are any pending callbacks. If so, it dequeues the callbacks one by one and pushes them onto the call stack to be executed.
The Call Stack
The call stack is a data structure that records where in the program we are, so we know how to get back to a point when a function returns. When a script calls a function, it is pushed onto the call stack. When that function returns, it is popped back off the stack.
Message Queue
The message queue is where callbacks are enqueued when an asynchronous event like a network request occurs. These callbacks sit in the queue until the call stack is empty, then the event loop pushes them one by one to the call stack to execute.
Asynchronous vs. Synchronous
Synchronous code is executed from top to bottom, one at a time. Asynchronous code is code that is executed at a later point in time. An example is when we make an AJAX request to a server - we provide a callback to handle the response but the code keeps executing while waiting for the server to respond. This is where the event loop kicks in - the callback gets enqueued and only executed once the call stack is empty.
Event-Driven Architecture
Event-driven architecture enables us to write code that reacts to events as they happen. When an event like a user clicking a button occurs, the browser adds the event callback to the message queue, allowing JavaScript to execute other tasks before that event is processed. This results in a non-blocking architecture that keeps the browser responsive and performant.
Summary
In summary, the JavaScript event loop together with concepts like the call stack, message queue, and event-driven architecture, allows JavaScript to handle concurrent operations and create responsive and performant applications. Understanding how the event loop works is key to writing efficient and well-structured JavaScript code.