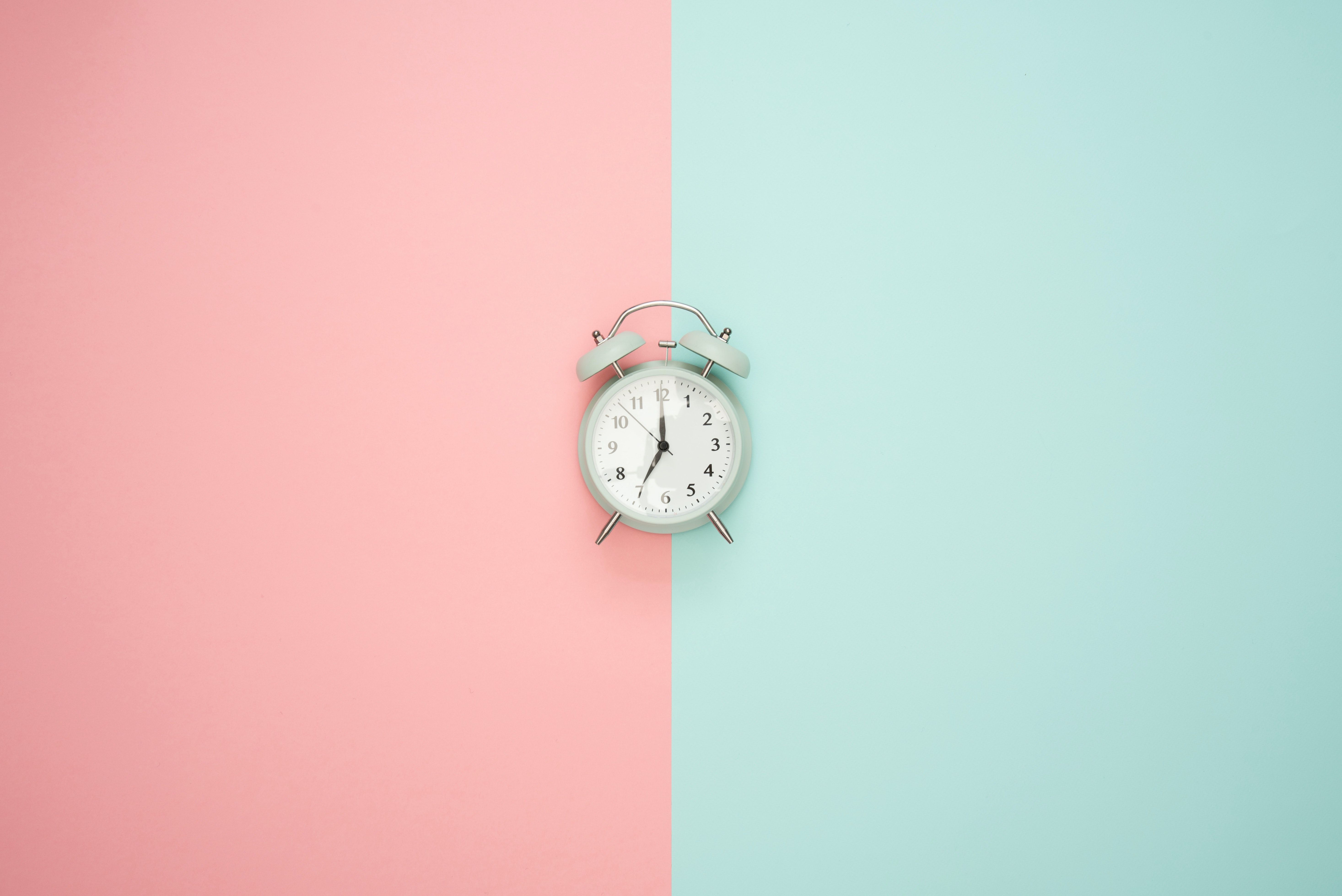
How To Create React and Tailwind project with Vite
Vite is the next generation front-end tooling. Vite is new bundler like webpack, Rollup, Parcel, or Snowpack but it is really fast. Vite is not like traditional bundler, it leverages the native esm which then can be dynamically served as the browser needs it. If you want to know more why Vite was create, please read this guide.
React and Tailwind
React is one of the popular JavaScript framework. I love creating my project with React and with React Hooks, Suspense and other tools that come with it, React is my number one framework / library for front-end projects.
Tailwind CSS, a styling framework that focus on utility classes is also one of my go to CSS framework to start new project. It is very simple and yet powerful to design your layout.
So without further ado, let's do this!
Create Vite and React based project
Before we dive into the code, please make sure you have Node >= 12 or Vite won't work.
First we need to create our project folder based on Vite. I name my app: vite-react. You can name it anything you like.
npm init @vitejs/app <app-name># oryarn create @vitejs/app <app-name>
Then all you need to do is choose the template you like. In this case we choose react and then we can go to next step which is installing the tailwind dependencies.
Installing Tailwind
Using tailwind with Vite is a breeze. It is very easy and fast to setup compare to create-react-app.
All we need to do is install tailwind dependencies:
npm install tailwindcss@latest postcss@latest autoprefixer@latest# oryarn add tailwindcss@latest postcss@latest autoprefixer@latest
Later we need to create postcss.config.js in our root folder. Just like in Tailwind installation guide.
module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, }}
If you like to customize your installation you can also create it via Tailwind CLI. I highly recommended this since we need to purge Tailwind unused class later.
npx tailwindcss init
Next, add Tailwind directives in our index.css
@tailwind base;@tailwind components;@tailwind utilities;
OK, that's it. Now you can use Tailwind in your React project. Really simple right? Compare it how to add Tailwind with create-react-app, I never remember how to set it up properly :)
Up and running...
Before we start our development server, let's change some code in our App.js first so we can see the difference.
// Line 12<p className="text-lg text-green-400">Hello Vite + React!</p>
Last thing we need is to install all the dependencies and run it:
# at root of your projectyarnyarn dev
Open your browser and go to localhost:3000, and voila... you made it. Congrats!
Conclusion
Vite makes creating React project with Tailwind or any other framework fast. As your code getting larger and larger, fast development experience is making your life easier, stress less, and fun.
Oh, I almost forgot, if you try to build it, it will be fast too. But the CSS bundling is big due to Tailwind nature, so don't forget to purge it in you tailwind.config.js like this:
module.exports = { purge: [ './src/**/*.html', './src/**/*.js', './src/**/*.jsx', ], darkMode: false, // or 'media' or 'class' theme: { extend: {}, }, variants: { extend: {}, }, plugins: [],}
Purging your unused CSS class will reduce the build CSS file significantly so now you can use it for production. Grab the source code at github.
Well, that's it folks until then, say hi to me in Twitter.
Ciao!