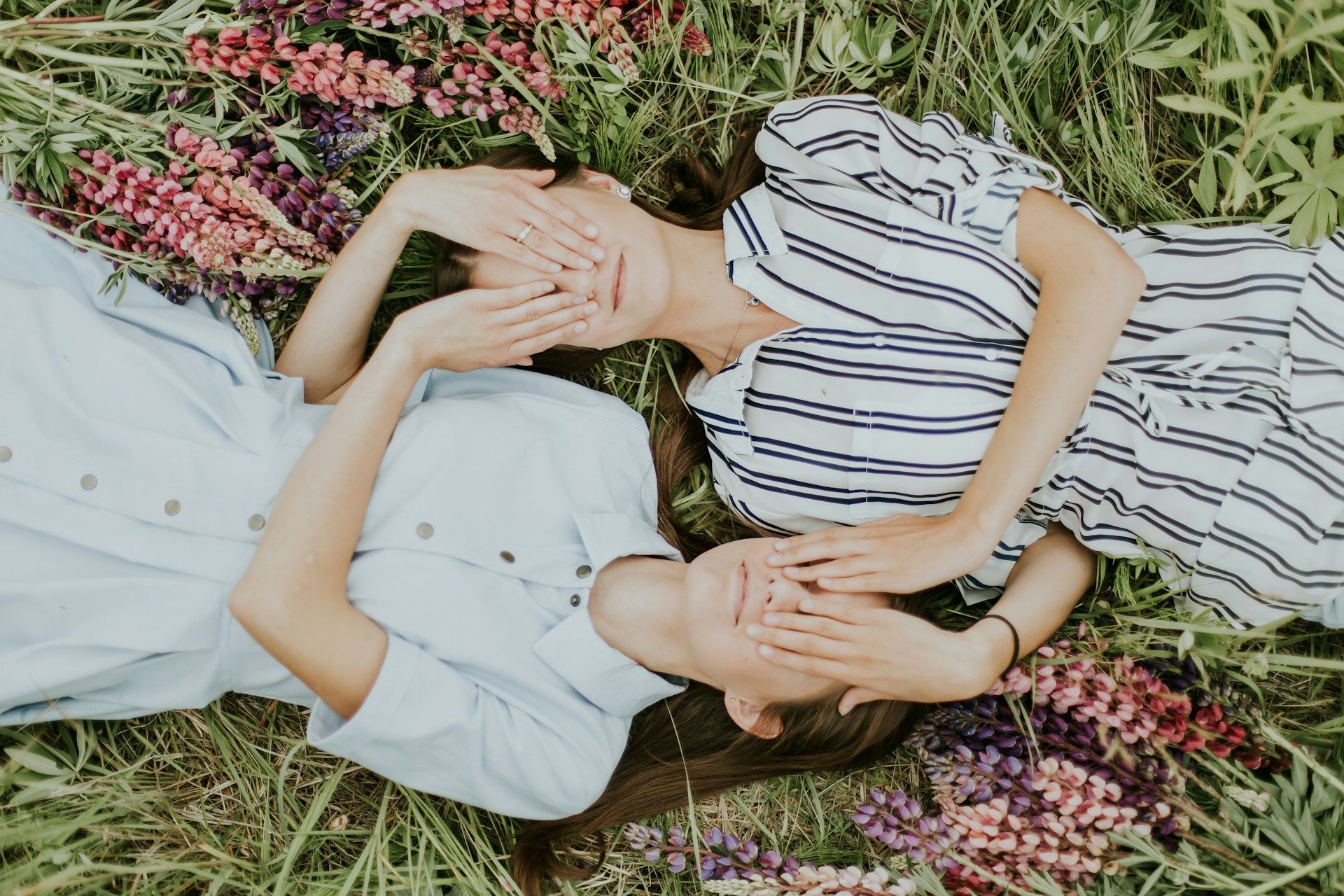
React Components vs Regular Functions: A Guide for New Developers
TLDR: create a functional component if you return JSX, otherwise create a regular function.
Ever felt like you're lost in the React jungle? You're not alone! As someone who's worked with fresh-faced developers straight out of college or 20-hour YouTube coding boot camps, I've seen the confusion firsthand. Let's clear the air and make React as easy as pie!
The React Revelation: It's Just JavaScript (With a Twist)
Here's the secret sauce: React is essentially JavaScript with a sprinkle of JSX magic. Mind-blowing, right? Let's dive into an example:
function Home({honey}) { return <h1>I'm Home {honey}!</h1>}
Looks simple, doesn't it? But this simplicity is where the plot thickens...
The Case of the Mysterious Function
Now, what if we wrote it like this?
function home({honey}) { return <h1>I'm Home {honey}</h1>}
Is it wrong? Nope! But here's the kicker.
Cue dramatic music
It's not a React component
The Capital Case Conundrum
React has its own set of rules, one of which is that component names must start with a capital letter. So, function Home is a full-fledged React component, while function home is just a regular JavaScript function.
But wait, there's more! Let's break down the differences:
- React components inherit React superpowers, like Hooks and custom hooks.
- Regular functions are hook-less (no superpowers here, folks).
- You summon a React component like this: <Home honey={'Suzy'} />
- Don't call a React component directly `Home({honey:'Suzy'})`.
- A regular function gets called the old-fashioned way: home({honey: 'Suzy'})
- React components can use cool React features like React.memo(Home), but regular functions are left out in the cold.
The Render Function Trap
This usually happens when refactoring the code, especially the `render` function. We can find the render function a lot in the React Class Component:
class Count extends React.Component { renderList = (lists) => { return lists.map(list => { return <li key={list.id}>{list.label}</li>; }) };
render() { return ( <main> <div> <ul> {renderList(lists)} </ul> </div> </main> ); }}
This is fine, but there are times when we need to optimize it because maybe we want to render a lot of lists. So how will you do it?
This is where I found that new React developer got confused. They thought that everything should be a function and had no idea what is the differences between regular function and React Functional component. New developer will put it outside of class component and that is it.
Actually, that is fine too, but we can't really optimize it. So there are a couple of ways to optimize the function, first, we can wrap it using React.memo and move the function outside the class component. Here is how I do it:
const RenderList = React.memo(({lists}) => { return lists.map(list => { return <li key={list.id}>{list.label}</li>; }) });
// and call it like this return ( <ul> <RenderList lists={lists} /> <ul> )
Remember, when working with React you need to uppercase your React Component name, or is just become a regular function.
Second, if you are using React Hooks, you could wrap it inside React.useCallback and set the lists in the array dependency.
const renderList = React.useCallback(() => { return lists.map(list => { return <li key={list.id}>{list.label}</li> })}, [lists])
The React Golden Rule
Here's a simple rule to live by: If you're returning JSX, create a functional component. Otherwise, stick to a regular function. It's that easy!
Why I Love React (and You Should Too)
React's beauty lies in its closeness to JavaScript. The only real difference? It can return JSX (or even Pug, if you're feeling adventurous).
Remember, in the world of React, capitalize your components, and may the hooks be ever in your favor!
That's all for now folks!
Next, I'll talk about my journey mixing Elixir...
The Phoenix is about to rise...